SOAP API¶
The shortcut SOAP stands for Simple Object Access Protocol. Most current programming languages support SOAP. Furthermore LiveConfig provides a WSDL file (Web Service Description Language) for automatic detection of all available methods and data types for a seamless integration into own applications.
The communication for SOAP access is done using XML message and “normal” HTTP(s) connections. For example, the invocation of the SOAP method TestSayHello looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<SOAP-ENV:Envelope xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:ns1="urn:LiveConfig">
<SOAP-ENV:Body>
<ns1:TestSayHello>
<ns1:auth>
<ns1:login>admin</ns1:login>
<ns1:timestamp>2009-12-22T19:20:59.000Z</ns1:timestamp>
<ns1:token>7juGMfg7N/F1G4t+S7XW99nRidg=</ns1:token>
</ns1:auth>
<ns1:firstname>Manfred</ns1:firstname>
<ns1:lastname>Mustermann</ns1:lastname>
</ns1:TestSayHello>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
On a successful invocation the response looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<SOAP-ENV:Envelope xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<SOAP-ENV:Body>
<TestSayHelloResponse xmlns="urn:LiveConfig">
<greeting>Hello, Manfred Mustermann</greeting>
</TestSayHelloResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
If an error occured while processing the request, a SOAP exception containing an appropriate error message is generated. For instance the exception because of an expired (invalid) timestamp looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<SOAP-ENV:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema"
SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/">
<SOAP-ENV:Body>
<SOAP-ENV:Fault>
<faultcode>SOAP-ENV:Server</faultcode>
<faultstring>Authentication timestamp too far in past or future</faultstring>
</SOAP-ENV:Fault>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
Prerequisites¶
The SOAP API of LiveConfig is accessible at the URL /liveconfig/soap
. SOAP calls must be encoded in document/literal style (not in RPC/encoded style!).
You also need
-
a LiveConfig Standard® or LiveConfig® Business license (the SOAP API is not available with Basic or Light license)
-
access to the LiveConfig®
admin
account -
having configured a SOAP password for the
admin
account (see next section)
Authentication¶
All SOAP methods require the submission of an auth
parameter, which contains the user name, a timestamp and the SOAP token. The token is a base64-encoded HMAC-SHA1 checksum on the string “LiveConfig”, the user name, the invoked method name and a time stamp; the SOAP password is used as key.
To set the SOAP password, run liveconfig --init
and set the environment variable LCINITSOAP
to your desired password:
#> LCINITSOAP="SoAp_pAsSwOrD" liveconfig --init
This example shows the creation of the auth
parameter in PHP:
<?php
$user = 'admin'; # user name
$pass = 'PaSsWoRt'; # SOAP password
$func = 'TestSayHello'; # SOAP method to call
# create timestamp, e.g. '2009-12-07T11:05:00.000Z'
$ts = gmdate("Y-m-d") . "T" . gmdate("H:i:s") . ".000Z";
# generate token:
$token = base64_encode(hash_hmac('sha1',
'LiveConfig' . $user . $func . $ts,
$pass,
true
)
);
# generate "auth" parameter:
$auth = array('login' => $user,
'timestamp' => $ts,
'token' => $token
);
?>
The timestamp in the auth
parameter must not differ more than 15 minutes from the LiveConfig server - otherwise you get an error message. The timestamp has to be given in UTC/GMT timezone.
Running SOAP methods with customer permissions
You can call all SOAP methods also with the permissions of any customer. This can be useful when working e.g. with reseller accounts.
In this case, the (encoded) customer ID needs to be added before hashing, and the field customer
be added to the auth
parameter.
Note
the customer with whom a function is to be called must also be authorized for the respective function.
I.e. you can not create additional customers when the user you’re working as has no reseller permissions.
Example in PHP how to run a SOAP method as a specific customer:
<?php
$user = 'admin'; # user name
$pass = 'PaSsWoRt'; # SOAP password
$func = 'TestSayHello'; # SOAP method to call
$cust = 'c9R4hQp.T-rF'; # encoded customer id
# create timestamp, e.g. '2009-12-07T11:05:00.000Z'
$ts = gmdate("Y-m-d") . "T" . gmdate("H:i:s") . ".000Z";
# generate token:
$token = base64_encode(hash_hmac('sha1',
'LiveConfig' . $user . $func . $ts . $cust,
$pass,
true
)
);
# generate "auth" parameter:
$auth = array('login' => $user,
'timestamp' => $ts,
'token' => $token,
'customer' => $cust
);
?>
WSDL Description¶
LiveConfig provides a WSDL description of all permitted methods and accompanying data types for a particular user. For this, append ?wsdl
as well as the user name and the SOAP password to the SOAP URL.
The following example shows the creation of a SOAP client in PHP based on a WSDL file:
<?php
$user = 'admin'; # User name
$pass = 'PaSsWoRt'; # SOAP password
# Construct WSDL URL
$url = 'https://your.liveconfig.server:8443/liveconfig/soap'
.'?wsdl' # request WSDL
.'&l=' . urlencode($user)
.'&p=' . urlencode($pass);
# Create SOAP client
$client = new SoapClient($url,
array('style' => SOAP_DOCUMENT,
'use' => SOAP_LITERAL,
)
);
?>
Caution
Currently the SOAP password for the WSDL request is appended unencrypted to the WSDL URL. The transmission to the server though is encrypted using the HTTPS protocol and in the access_log from LiveConfig the password is masked. In later versions of LiveConfig the use of a password hash is planned. But as long as some programming languages (e.g. the WebService Wizzard from C#) expect the input of a WSDL URL, a manual hash calculation isn’t practical.
Example Code¶
In this section you find some examples for using the SOAP API with common program languages.
PHP¶
PHP has native SOAP support. The only prerequisite is that the SOAP module is loaded (when compiling PHP by yourself, use the option --enable-soap
). If SOAP support is enabled can be seen in the output of phpinfo()
:
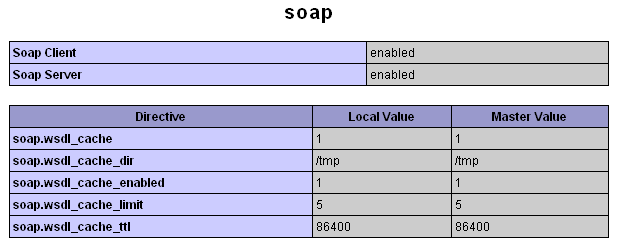
The following example program shows all necessary steps to send a SOAP request to LiveConfig:
<?php
# Configuration parameters:
$user = 'admin';
$pass = 'PaSsWoRd';
$url = 'https://your.liveconfig.server:8443/liveconfig/soap';
# Construct WSDL URL
$wsdl_url = $url
.'?wsdl'
.'&l=' . urlencode($user)
.'&p=' . urlencode($pass);
# Create SOAP client
$client = new SoapClient($wsdl_url,
array('style' => SOAP_DOCUMENT,
'use' => SOAP_LITERAL,
)
);
# Construct SOAP token:
$ts = gmdate("Y-m-d") . "T" . gmdate("H:i:s") . ".000Z";
$token = base64_encode(hash_hmac('sha1',
'LiveConfig' . $user . 'TestSayHello' . $ts,
$pass,
true
)
);
$auth = array('login' => $user,
'timestamp' => $ts,
'token' => $token);
$params = array('auth' => $auth,
'firstname' => 'John',
'lastname' => 'Doe');
try {
$response = $client->TestSayHello($params);
} catch (SoapFault $soapFault) {
die("Error while calling Web Service: " . $soapFault->faultstring . "\n");
}
echo "Response: " . $response->greeting . "\n";
?>
SOAP Reference¶
- ContactAdd
- ContactEdit
- ContactGet
- CustomerAdd
- CustomerDelete
- CustomerEdit
- CustomerGet
- HostingCronAdd
- HostingDCVCreate
- HostingDCVDelete
- HostingDatabaseAdd
- HostingDatabaseDelete
- HostingDomainAdd
- HostingFtpAdd
- HostingLookup
- HostingMailboxAdd
- HostingMailboxEdit
- HostingMailboxGet
- HostingPasswordPathAdd
- HostingPasswordUserAdd
- HostingPlanAdd
- HostingPlanGet
- HostingSubdomainAdd
- HostingSubdomainEdit
- HostingSubscriptionAdd
- HostingSubscriptionDelete
- HostingSubscriptionEdit
- HostingSubscriptionGet
- LiveConfigVersion
- SessionCheck
- SessionCreate
- TestSayHello
- UserAdd
- UserEdit
- UserGet